Today, each eCommerce store sells a wide range of products, and users are often confused and lost in the websites. In such scenarios, your business may suffer, and you will make fewer sales despite having all the products in stock. Moreover, customers do not like waiting in line to talk to a customer representative over a call, needing a quick solution to common questions. If you cannot cater to your customers quickly, you will often lose them to another eCommerce website that can do so.
The way out of such a dilemma is to have a chatbot that has extensive features. ChatGPT was released a few months back, and since then, it has taken the world by storm. Everyone is talking about its fantastic features. In this article, we will have a look at what ChatGPT is, what a chatbot is, and how you can build an eCommerce website with a chatbot feature using Python.
So let us get started by understanding chatbots.
What is a Chatbot?
A chatbot system uses conversational artificial intelligence to imitate a natural language discussion with any user via messaging applications, websites, or mobile apps. Such systems employ rule-based language applications to provide live chat capabilities and deliver real-time responses to users.
A chatbot has many different use cases, and it works well with eCommerce apps and websites. Creating a chatbot from scratch and training it for all the possible outcomes is a huge and time-consuming task. Moreover, such an approach to building chatbots is quite expensive, and you need a lot of experts. Instead, you can use a pre-trained natural language model to power your chatbots.
In the upcoming section, we will have a look at the latest language model that has had such a huge impact in recent months – GhatGPT.
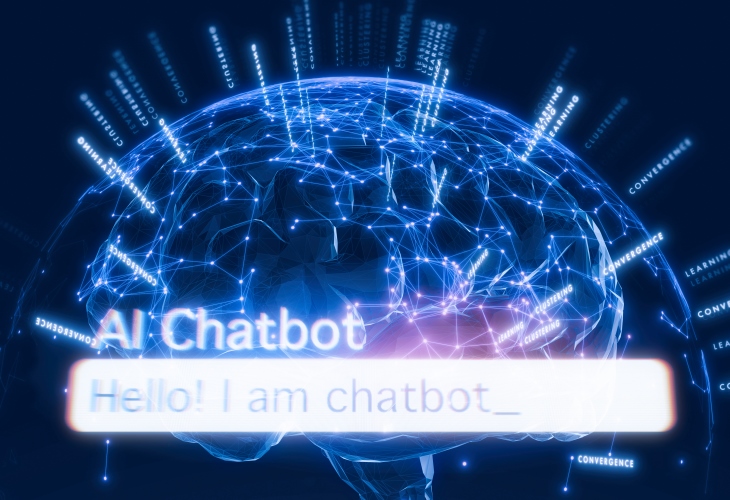
What is ChatGPT?
ChatGPT is an NLP (Natural Language Processing) solution based on AI technology that allows you to have humanlike discussions with the chatbot and much more. This language model can answer questions, aid you with chores, produce letters and articles, and even generate code.
This language model was created by OpenAI, an AI research company with a focus and expertise in developing AI products, and it has developed several products, such as OpenAI’s Dall-E 2, an art generator that can create pictures out of your descriptions.
Having understood what ChatGPT is, it is better to look at why you should use chatbots in your eCommerce website.
Why Use Chatbots in eCommerce Websites?
1. Personalized Servicing to Customers
Personalization is essential in e-commerce, and chatbots are an excellent technique to establish a better, more relevant connection.
Chatbots may be used to collect data about the visitors to your eCommerce website and utilize that data to generate better product suggestions and recommendations. Understanding consumer queries, requirements, and preferences may assist you in personalizing product pages and increasing customer loyalty to your company.
Furthermore, clever chatbots may warn clients when their favorite things are out of stock and propose other products based on their tastes, all while notifying them of their projected delivery date and time as well.
2. Shift Customer Support Executive to L2 Operations
When you have a bustling eCommerce store, it is natural that your customers will have many queries, and they will need assistance.
To provide such assistance at a large scale, you need to hire many different customer support executives, and they all will be doing the same repetitive tasks. Such an approach is not efficient or feasible beyond a certain point.
That is where chatbots come into the picture. You can create and train chatbots for specific queries, and they can keep handling such queries forever.
Moreover, you can develop a single chatbot and deploy it across multiple websites, which will help you reduce the dependency and workloads of customer executives.
When the workload of your customer support executives decreases at the lower level, you can shift them to solve level 2 escalation queries. The faster you solve such queries, the better it is for your brand.
3. Fast and Cost-Effective Customer Support
When you go global, you cannot say that your work hours are over, and you cannot serve customer queries. A large number of customers require instant solutions to their issues, and if that is not available, they often head over to another eCommerce store.
Setting up human customer support executives for 24/7 quick solutions is not a feasible option either. You cannot scale such an operation as you grow your business; it is also quite costly. Chatbots are the only way that can provide you with instant customer support feature at significantly lesser prices.
AI chatbots can help in solving common customer requests quickly, and they can also learn from the conversations to provide better customer support each time. Moreover, there is no downtime in chatbots. And once they are installed, they can serve customer needs as and when they come.
4. Set Up a Knowledge Base
Scrolling through documents or reading documentation pages is a thing of the past. Today, there are very few customers who prefer that way to understand or gain information regarding anything. You cannot expect your customers to review entire documents before making an eCommerce transaction, as there are easier ways to educate them. Chatbots act as a great knowledge base, and when you program them and keep them updated with new data every time, your chatbots can help more users in understanding the products.
When your customers can understand the products quickly, the chances of dropping a transaction decrease significantly.
5. Collect and Act on Feedback
One of the best and tested ways to improve your business is by collecting and acting on feedback. If you have an eCommerce store where you have a feedback form, you are likely to get very few responses. On the other hand, having a chatbot collect feedback from visitors has a better success rate.
Chatbot can complete conversations with visitors and, in the end, ask for feedback for the conversation.
This way, the chatbot can understand whether it served the customer correctly or not. Even if you do not develop a self-learning chatbot, you can use that feedback data and understand where your services lag. Once you know the areas of improvement, it becomes much easier to improve your services without doing unnecessary work.
By now, you know why chatbots are important for an eCommerce website. Now, you might be excited to build your own chatbot, and that is what the next section is all about.
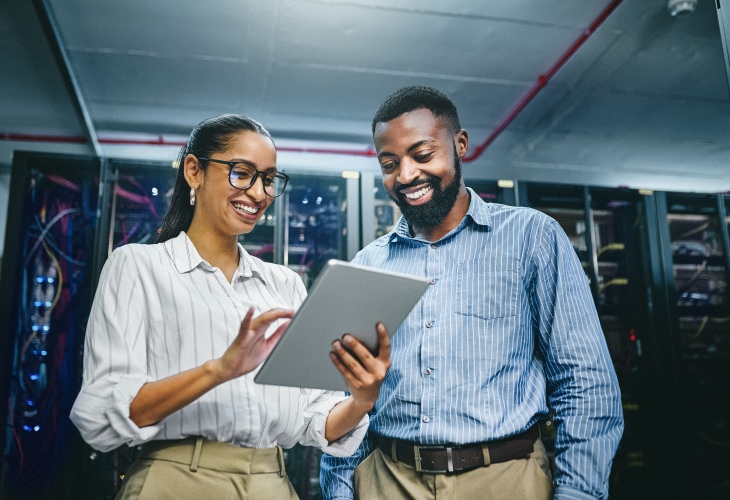
How to Make an eCommerce Website Chatbot with Python and ChatGPT?
In this section, we will have a look at building an eCommerce chatbot using Python and ChatGPT. So let us start with setting up the environment.
To get started on your chatbot development, you will need Python’s Flask web framework. Moreover, we will also use ChatGPT’s PyTorch implementation in this project.
If you do not have Flask and PyTorch installed in your system, use the below commands in your terminal to install the packages.
- Pip install flask
- Pip install torch
- Pip install transformers
Once you run these commands, the required packages will be installed in your system, and you can proceed. Create a Flask project by creating a new Python file and adding the below code in that.
- from flask import Flask, request, jsonify
- import torch
- from transformers import GPT2Tokenizer, GPT2LMHeadModel
Once you have imported the required packages, it is time to use the pre-trained ChatGPT model and create a chatbot for your eCommerce website. Use the below code to select the transformer and initialize the model.
- tokenizer = GPT2Tokenizer.from_pretrained(‘gpt2’)
- model = GPT2LMHeadModel.from_pretrained(‘gpt2’, pad_token_id=tokenizer.eos_token_id)
Now that your model is initialized, you need a function that can be called to use the model and return a response to the users. Copy the upcoming Python function and paste it into your file to accept queries and return responses.
def generate_response(prompt):
input_ids = tokenizer.encode(prompt, return_tensors=’pt’)
output = model.generate(input_ids, max_length=50, num_return_sequences=1, no_repeat_ngram_size=2, early_stopping=True)
response = tokenizer.decode(output[0], skip_special_ tokens=True)
return response
The above function will take prompts from the user, and it will process the prompt using the ChatGPT model that you initialized earlier. Moreover, it will also return the generated response to the calling function.
To use this function on your website, you need to develop the Flask app, which is pretty simple. Use the below code to create a Flask app and call the function to return helpful responses to users.
app = Flask(name)
@app.route(‘/’)
def home():
return ‘Welcome to our e-commerce website!’
@app.route(‘/chatbot’, methods=[‘POST’])
def chatbot():
data = request.json
prompt = data[‘prompt’]
response = generate_response(prompt)
return jsonify({‘response’: response})
There are two endpoints/URLs that can be invoked by a visitor. In the above code, the user will have to send a POST request on the /chatbot endpoint with a prompt to get a response from your chatbot. To test the chatbot and get a response, you can use the below code.
import requests
response = requests.post(‘http://localhost:5000/chatbot’, json={‘prompt’: ‘What products do you sell?’})
print(response.json()[‘response’])
The above code will send a request to your /chatbot endpoint with a prompt, and the route will return the response by invoking the GPT model function.
Conclusion
Coming to an end, you have finally understood how to create a chatbot and the latest ChatGPT language model. You can retrain this model with data that is relevant to your eCommerce store to support more personalized conversations and services on the platform.